Solved: "Class Extends Undefined" Error in JavaScript
JavaScript errors can sometimes be cryptic, and one that often confuses developers is:
TypeError: Class extends value undefined is not a function or null
If you've encountered this error, don’t worry! In this post, we'll break it down and explore ways to fix it.
What Causes This Error?
This error typically happens when a JavaScript class attempts to extend something that is undefined
or null
. Here are the most common reasons:
Video Tutorial:
1. Incorrect Import or Export
If you're using ES6 modules, a misconfigured import or export can lead to an undefined value.
Example:
// File: myClass.js
export class MyClass {}
// File: main.js
import { MyClas } from './myClass'; // Typo! MyClas instead of MyClass
class MySubClass extends MyClas {} // ❌ Error here!
Solution:
- Double-check your import/export statements.
- Ensure the imported module actually exists.
- Fix typos in import names.
2. Circular Dependencies
Circular dependencies happen when two modules import each other in a loop. This can cause one module to be undefined
when it's imported.
Example:
// File: A.js
import { B } from './B';
export class A extends B {} // ❌ B is undefined!
// File: B.js
import { A } from './A';
export class B extends A {}
Solution:
- Refactor your code to remove circular dependencies.
- Use dependency injection or move shared logic to a separate module.
3. Missing or Incorrect Dependencies
If you’re extending a class from a library that isn't installed or correctly imported, you might see this error.
Example:
// Assuming React isn't imported
class MyComponent extends Component {} // ❌ Error
Solution:
- Make sure all required dependencies are installed (npm install <package>).
- Import the required class correctly:
import React, { Component } from 'react';
class MyComponent extends Component {} ✅
4. Class Is Not Defined Before Use
If a class is referenced before it is defined, JavaScript will throw this error.
Example:
class MySubClass extends MyParentClass {} // ❌ MyParentClass isn't defined yet!
class MyParentClass {}
Solution:
- Ensure the parent class is defined before the subclass.
- Move the class definition above its usage.
Still not fixed?
If you've implemented all the above mentioned solution, but the error persists - I'd love to help you out. Shoot me an email.
Email: dhanuskkar@cybermindworks.com (response time within 3hrs)
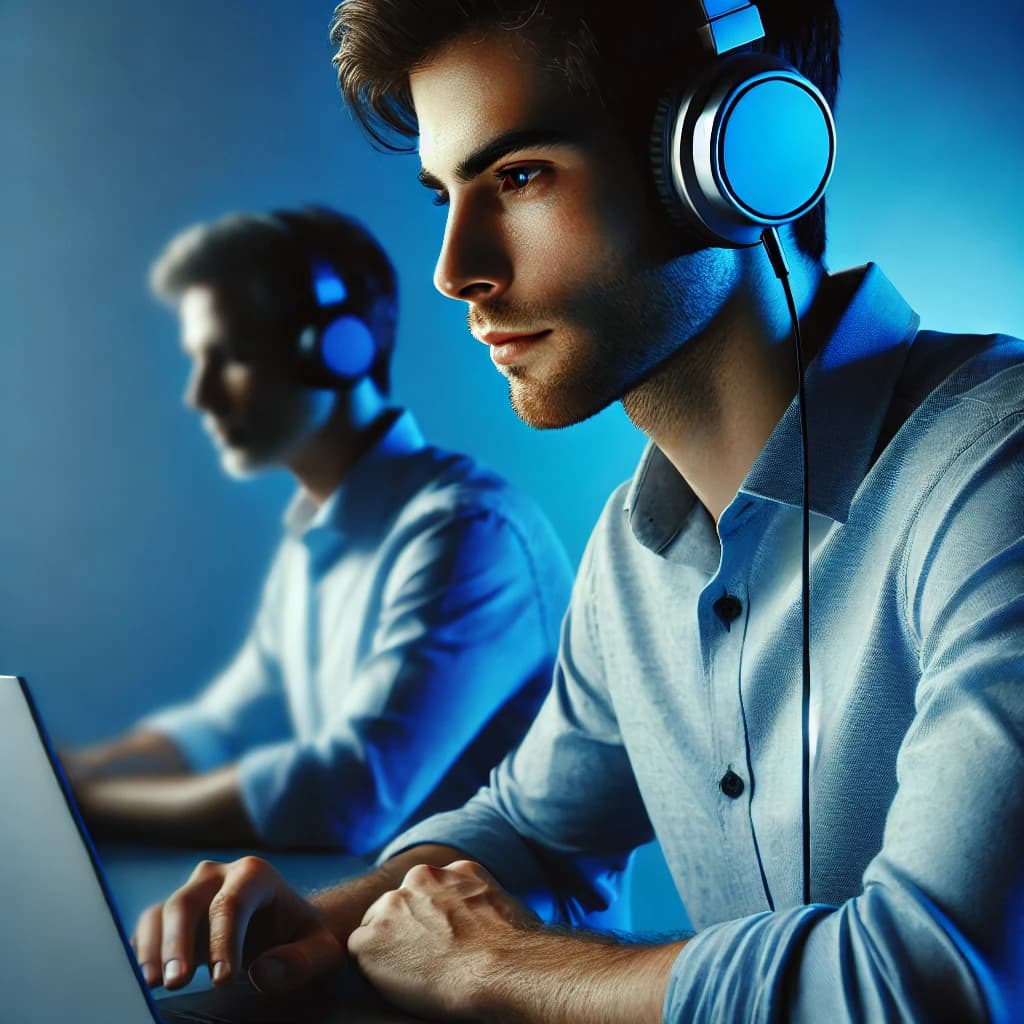