Socket.IO is a JavaScript library that facilitates real-time, bidirectional communication between clients and servers. Built on top of WebSockets, it provides robust fallback mechanisms for compatibility across various network and browser environments.
Why Use Socket.IO?
- Real-Time Communication: Perfect for chat applications, notifications, and collaborative tools.
- Cross-Browser Support: Automatically selects the best transport method.
- Event-Driven Architecture: Simplifies communication using custom events.
- Scalable: Supports namespaces, rooms, and broadcasting for large-scale systems.
What is Socket.IO?
Socket.IO bridges the gap between client-server communication by providing a persistent connection. Unlike traditional HTTP, which is stateless, Socket.IO maintains a stateful connection, enabling real-time interactions.
Key Use Cases
- Chat Applications: Enable real-time messaging across multiple users.
- Live Dashboards: Stream updates for analytics or notifications.
- Gaming Applications: Deliver low-latency updates for multiplayer games.
- Collaborative Tools: Power shared whiteboards or live document editing.
- Live Streaming: Provide seamless audio, video, or data streams.
Socket.IO Transport Methods
Socket.IO employs multiple transport protocols to ensure reliable communication:
1. WebSockets
- Use Case: Low-latency bidirectional communication for gaming or chat.
- Advantage: Highly efficient with minimal overhead.
- Limitation: Requires full WebSocket support in the browser and server.
2. HTTP Long Polling
- Use Case: Works as a fallback in restricted environments.
- Advantage: Compatible with legacy systems.
- Limitation: Higher latency and server load.
3. HTTP Streaming
- Use Case: Ideal for continuous, one-way updates like news tickers.
- Advantage: Efficient for broadcasting.
- Limitation: No bidirectional communication.
How to Implement Socket.IO
Basic Server Example
const { createServer } = require('http');
const { Server } = require('socket.io');
const httpServer = createServer();
const io = new Server(httpServer, {
cors: {
origin: "*",
methods: ["GET", "POST"]
}
});
io.on('connection', (socket) => {
console.log(`Connected: ${socket.id}`);
socket.on('message', (data) => {
console.log(data);
io.emit('message', data); // Broadcast message
});
socket.on('disconnect', () => {
console.log(`Disconnected: ${socket.id}`);
});
});
httpServer.listen(3000, () => {
console.log('Server running on port 3000');
});
Client Example
<!DOCTYPE html>
<html>
<head>
<title>Socket.IO Example</title>
<script src="<https://cdn.socket.io/4.5.0/socket.io.min.js>"></script>
</head>
<body>
<input id="message" placeholder="Type a message..." />
<button id="send">Send</button>
<ul id="messages"></ul>
<script>
const socket = io('<http://localhost:3000>');
document.getElementById('send').onclick = () => {
const msg = document.getElementById('message').value;
socket.emit('message', msg);
};
socket.on('message', (msg) => {
const li = document.createElement('li');
li.textContent = msg;
document.getElementById('messages').appendChild(li);
});
</script>
</body>
</html>
Advanced Features in Socket.IO
1. Room-Based Communication
Group users into rooms for targeted messaging:
socket.join('room1');
io.to('room1').emit('message', 'Hello Room 1!');
2. Acknowledgements
Ensure reliability with server-client acknowledgements:
socket.emit('event', { data: 'Hello' }, (response) => {
console.log('Acknowledged:', response);
});
3. Middleware for Authentication
Authenticate users before establishing a connection:
io.use((socket, next) => {
const token = socket.handshake.auth.token;
if (isValidToken(token)) {
next();
} else {
next(new Error('Authentication error'));
}
});
Socket.IO Admin UI
The Socket.IO Admin UI is a real-time dashboard for monitoring and debugging Socket.IO applications. It provides critical insights into active connections, emitted events, and namespace activities, making it a valuable tool for developers managing complex real-time systems.
Setting Up the Admin UI
1. Install the Admin UI Package
npm install @socket.io/admin-ui
2. Configure the Admin UI in the Backend
Integrate the Admin UI into your Socket.IO server:
const { createServer } = require('http');
const { Server } = require('socket.io');
const { instrument } = require('@socket.io/admin-ui');
const httpServer = createServer();
const io = new Server(httpServer, {
cors: {
origin: ["<https://admin.socket.io>"], // Admin UI origin
credentials: true,
},
});
// Setup Admin UI
instrument(io, {
auth: false, // Disable authentication for testing
});
io.on('connection', (socket) => {
console.log(`Connected: ${socket.id}`);
});
httpServer.listen(3000, () => {
console.log('Server running on port 3000');
});
3. Access the Admin UI
- Open https://admin.socket.io in your browser.
- Connect to your server using its URL.
Admin UI Features
- Monitor Connections: Track active clients and their activity.
- View Events: Inspect emitted and received events in real-time.
- Manage Namespaces: Debug and configure custom namespaces.
- Room Insights: Check the distribution of users across rooms.
Pros and Cons of Socket.IO
Pros
- High-level API for real-time communication.
- Cross-browser and network compatibility.
- Built-in scalability with namespaces and rooms.
Cons
- Overhead compared to raw WebSockets.
- Requires additional setup for distributed systems.
Conclusion
Socket.IO is an excellent library for building real-time applications, offering simplicity, scalability, and rich features. The introduction of the Admin UI enhances its usability by providing developers with a comprehensive dashboard for monitoring and debugging. Whether you’re building chat apps, dashboards, or collaborative tools, Socket.IO is a robust solution to simplify real-time communication.
Explore how we can help build your next real-time application. Contact Cybermindworks today!
Further Reading
- Socket.IO Official Documentation
- WebSockets Protocol
- Scaling Socket.IO with Redis
- Best Practices for Real-Time Applications
About Rishaba Priyan
Rishaba Priyan: Frontend Developer | Crafting Seamless User Experiences
At CyberMind Works, Rishaba Priyan excels as a Frontend Developer, specializing in creating intuitive and engaging user interfaces. Leveraging his expertise in technologies like Next.js, Rishaba focuses on delivering seamless digital experiences that blend aesthetics with functionality.
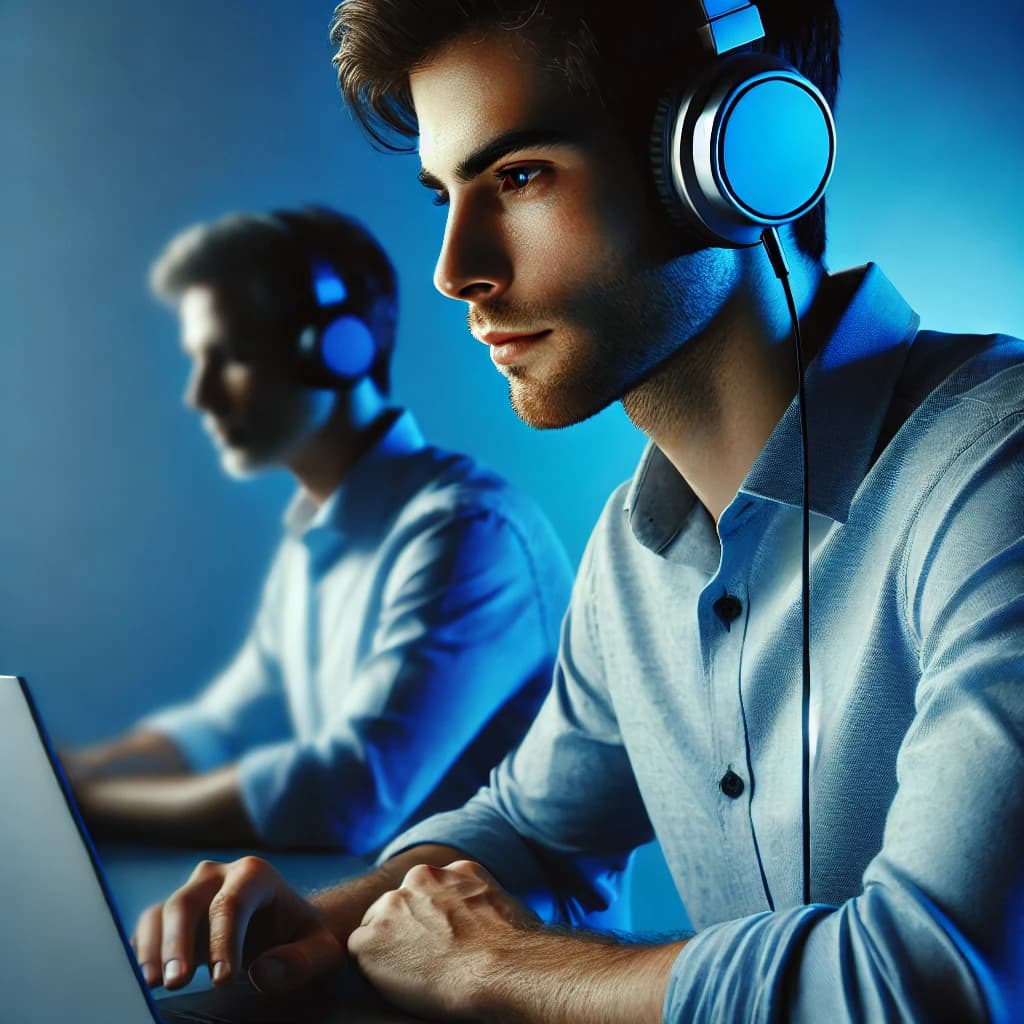