Microsoft Teams offers two primary ways to schedule meetings: through the Calendar API and the OnlineMeeting feature. While the Calendar API is tightly integrated with Outlook, creating and updating events automatically in your Outlook calendar, the OnlineMeeting feature is more flexible. It focuses solely on facilitating online meetings, making it an excellent choice for applications that need to schedule meetings independently of any calendar type.
In this guide, we will delve into the OnlineMeeting feature, providing step-by-step instructions on how to create, update, and delete meetings in Microsoft Teams using this powerful API.
Setting Up Your Application Registration
Before we dive into coding, we need to set up an application registration in Azure. This allows our application to interact with Microsoft Graph and leverage the OnlineMeeting feature.
Steps to Create a New App Registration
1. Navigate to App Registrations:
- Go to the Azure portal and find the App registrations service.

2. Create a New Registration:
- Click on New registration.
- Provide a name for your app and select the appropriate account type.
3. Manage Certificates & Secrets:
- After creating the registration, go to the Manage section and select Certificates & Secrets.
- Click on New client secret and copy the Secret Value and Secret ID. These will be needed later.

4. Add API Permissions:
- Navigate to API permissions and click on Add a permission.
- Select Microsoft Graph and choose the desired permission type: Delegated permissions or Application permissions.

Note: Application permissions work only on Web / Daemon type applications and require admin consent.
5. Grant Permissions:
- For scheduling meetings without a signed-in user, select the OnlineMeetings.ReadWrite.All permission.
- Click on Add permissions and ensure an admin grants consent for these permissions.
Configuring Application Access Policy
To allow your app to access cloud communications APIs with application permissions, you need to set up an application access policy.
1. Log in to Microsoft Teams via PowerShell:
- Open PowerShell and run:
Connect-MicrosoftTeams
2. Create a New Application Policy:
- Replace your_policy_name and your_application_id with your own values:
New-CsApplicationAccessPolicy -Identity your_policy_name -AppIds "your_application_id" -Description "Allow app to create meetings for user"
3. Grant Policy Access:
- To grant access to the entire tenant:
Grant-CsApplicationAccessPolicy -PolicyName your_policy_name -Global
- Or to a specific user:
Grant-CsApplicationAccessPolicy -PolicyName your_policy_name -Identity "user_object_id"
Creating, Updating, and Deleting Online Meetings
Now that our app is set up, let's explore how to create, update, and delete online meetings programmatically.
Creating a Meeting
To create a meeting, follow these steps:
1. Initialize the ConfidentialClientApplication:
private getClient() {
return new ConfidentialClientApplication({
auth: {
clientId: this.clientId,
authority: `https://login.microsoftonline.com/${this.tenantId}`,
clientSecret: this.clientSecret,
},
});
}
2. Get the Access Token:
private async getAccessToken() {
const clientCredentialRequest = {
scopes: ["https://graph.microsoft.com/.default"],
};
const response = await this.getClient().acquireTokenByClientCredential(clientCredentialRequest);
return response.accessToken;
}
3. Retrieve the User's Object ID:
private async getUserObjectId(accessToken: string, email: string): Promise<string> {
const client = Client.init({
authProvider: (done) => {
done(null, accessToken);
},
});
const user = await client.api(`/users/${email}`).get();
return user.id;
}
4. Create the Meeting:
async createMeeting() {
const accessToken = await this.getAccessToken();
const userObjectId = await this.getUserObjectId(accessToken, userEmail);
const client = Client.init({
authProvider: (done) => {
done(null, accessToken);
},
});
return client.api(`/users/${userObjectId}/onlineMeetings`).post({
startDateTime: startDate,
endDateTime: endDate,
subject: dto.title,
isOnlineMeeting: true,
});
}
Updating an Online Meeting
To update an online meeting, you can use the following code:
const accessToken = await this.getAccessToken();
const userObjectId = await this.getUserObjectId(
accessToken,
meeting.meetingTaker.email
);
const client = Client.init({
authProvider: (done) => {
done(null, accessToken);
},
});
client
.api(`/${userObjectId}/onlineMeetings/${meeting.platformMeetingId}`)
.update({
startDateTime: startDate,
endDateTime: endDate,
subject: meeting.title,
});
Delete an Online Meeting
To delete an online meeting, you can use the following code:
const accessToken = await this.getAccessToken();
const userId = await this.getUserObjectId(accessToken, userEmail);
const client = Client.init({
authProvider: (done) => {
done(null, accessToken);
},
});
await client
.api(`/users/${userId}/onlineMeetings/${platformMeetingId}`)
.delete();
Conclusion
This guide should help you get started with scheduling, updating, and deleting Microsoft Teams meetings using the OnlineMeeting feature. Remember to securely store your client secrets and other sensitive information related to your app registration.
About Rajeshwaran K
Rajeshwaran K is a Backend Developer at CyberMind Works. His expertise in developing robust and efficient server-side applications ensures the smooth functioning of complex systems. Rajeshwaran is dedicated to optimizing performance and reliability in his backend solutions.
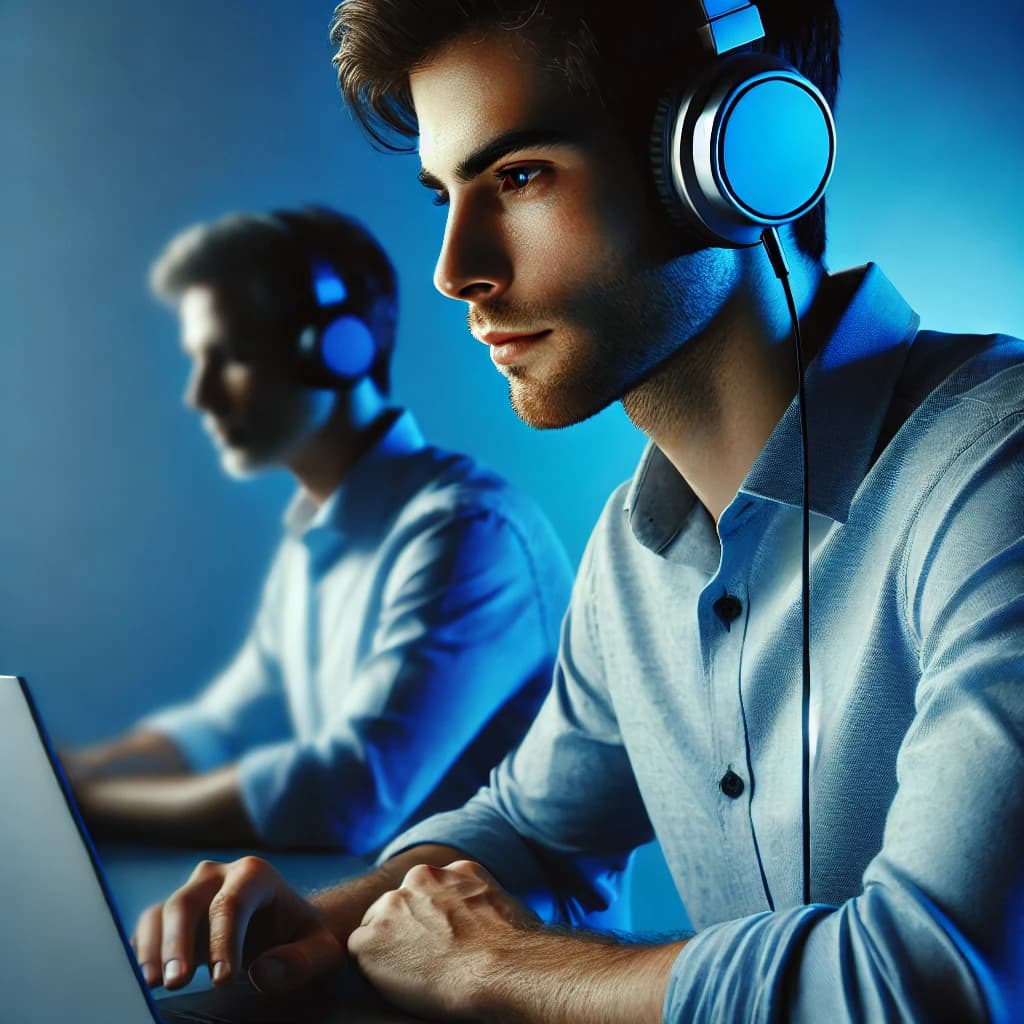