Drag-and-drop functionality is a powerful feature that enhances user interactivity in web applications. Whether it's file uploads, reordering lists, or moving items between containers, drag-and-drop offers intuitive ways for users to interact with content. In this guide, we’ll explore how drag-and-drop works, dive into real-world use cases, and provide code examples for implementation, including advanced scenarios with libraries like react-dnd
and @hello-pangea/dnd
.
How Drag-and-Drop Works in the Browser
The HTML5 Drag-and-Drop API provides the foundation for drag-and-drop interactions. By using event listeners and the DataTransfer
object, you can define drag sources and drop targets.
Key Events in Drag-and-Drop
Event | Purpose |
---|---|
dragstart | Fired when dragging begins; sets data to be transferred. |
dragover | Triggered when the draggable hovers over a valid drop target. |
drop | Triggered when the draggable is dropped on a target. |
dragend | Fired when the drag operation is complete, regardless of success. |
Basic Drag-and-Drop Implementation
Here’s an example of implementing a simple drag-and-drop feature:
<div id="drag-source" draggable="true" style="cursor: grab; padding: 10px; background: lightblue; width: 100px;">
Drag me
</div>
<div id="drop-target" style="border: 2px dashed #ccc; padding: 20px; margin-top: 20px;">
Drop here
</div>
<script>
const dragSource = document.getElementById('drag-source');
const dropTarget = document.getElementById('drop-target');
dragSource.addEventListener('dragstart', (event) => {
event.dataTransfer.setData('text/plain', 'Dragged Content');
dragSource.style.opacity = '0.5';
});
dropTarget.addEventListener('dragover', (event) => {
event.preventDefault(); // Allow drop
dropTarget.style.backgroundColor = '#e0f7fa';
});
dropTarget.addEventListener('drop', (event) => {
event.preventDefault();
const data = event.dataTransfer.getData('text/plain');
dropTarget.innerHTML = `Dropped content: ${data}`;
dropTarget.style.backgroundColor = '#fff';
});
dragSource.addEventListener('dragend', () => {
dragSource.style.opacity = '1';
});
</script>
What happens here:
- Drag Source: Initiates the drag event and sets transferable data.
- Drop Target: Accepts the dropped data and performs an action.
Popular Drag-and-Drop Use Cases
1. File Uploads
Allow users to upload files by dragging and dropping them into a designated area.
<div id="file-dropzone" style="border: 2px dashed #ccc; padding: 30px; text-align: center;">
Drag and drop files here
</div>
<script>
const dropzone = document.getElementById('file-dropzone');
dropzone.addEventListener('dragover', (event) => {
event.preventDefault();
dropzone.style.backgroundColor = '#f0f8ff';
});
dropzone.addEventListener('drop', (event) => {
event.preventDefault();
dropzone.style.backgroundColor = '#fff';
const files = event.dataTransfer.files;
const fileNames = Array.from(files).map((file) => file.name).join(', ');
dropzone.textContent = `Files: ${fileNames}`;
console.log('Dropped files:', files);
});
</script>
2. Reordering Lists
This feature is commonly used in dashboards, task management tools, or sortable lists.
Using @hello-pangea/dnd
import React, { useState } from 'react';
import { DragDropContext, Droppable, Draggable } from '@hello-pangea/dnd';
const ReorderableList = () => {
const [items, setItems] = useState(['Task 1', 'Task 2', 'Task 3']);
const onDragEnd = (result) => {
if (!result.destination) return;
const reordered = Array.from(items);
const [movedItem] = reordered.splice(result.source.index, 1);
reordered.splice(result.destination.index, 0, movedItem);
setItems(reordered);
};
return (
<DragDropContext onDragEnd={onDragEnd}>
<Droppable droppableId="list">
{(provided) => (
<ul {...provided.droppableProps} ref={provided.innerRef}>
{items.map((item, index) => (
<Draggable key={item} draggableId={item} index={index}>
{(provided) => (
<li
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
style={{
padding: '10px',
border: '1px solid #ccc',
marginBottom: '5px',
}}
>
{item}
</li>
)}
</Draggable>
))}
{provided.placeholder}
</ul>
)}
</Droppable>
</DragDropContext>
);
};
export default ReorderableList;
3. Dragging Across Containers
Move items between containers, like tasks in a Kanban board.
Using react-dnd
import React from 'react';
import { DndProvider, useDrag, useDrop } from 'react-dnd';
import { HTML5Backend } from 'react-dnd-html5-backend';
const DraggableItem = ({ id, name }) => {
const [, drag] = useDrag(() => ({
type: 'ITEM',
item: { id },
}));
return (
<div ref={drag} style={{ padding: '10px', border: '1px solid #ccc', margin: '5px' }}>
{name}
</div>
);
};
const DroppableContainer = ({ onDrop }) => {
const [, drop] = useDrop(() => ({
accept: 'ITEM',
drop: (item) => onDrop(item.id),
}));
return (
<div ref={drop} style={{ border: '2px dashed #ccc', padding: '20px', minHeight: '100px' }}>
Drop items here
</div>
);
};
const App = () => {
const [items, setItems] = React.useState([
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
]);
const handleDrop = (id) => {
setItems((prev) => prev.filter((item) => item.id !== id));
};
return (
<DndProvider backend={HTML5Backend}>
<div style={{ display: 'flex', gap: '20px' }}>
<div>
{items.map((item) => (
<DraggableItem key={item.id} {...item} />
))}
</div>
<DroppableContainer onDrop={handleDrop} />
</div>
</DndProvider>
);
};
export default App;
Choosing the Right Library
Library Best For react-dnd
Complex multi-container drag-and-drop scenarios @hello-pangea/dnd
Simple reordering tasks primereact/tree
Hierarchical drag-and-drop (e.g., file trees)
Best Practices
- Optimize Performance: Minimize re-renders during drag events.
- Mobile Support: Use touch backends for drag-and-drop on mobile devices.
- Accessibility: Ensure drag-and-drop is navigable via keyboard and screen readers.
Further Reading
Drag-and-drop adds an extra layer of interactivity to your applications. With this guide, you’re now equipped to implement robust solutions tailored to your needs. Happy coding! 🚀
About Rishaba Priyan
Rishaba Priyan: Frontend Developer | Crafting Seamless User Experiences
At CyberMind Works, Rishaba Priyan excels as a Frontend Developer, specializing in creating intuitive and engaging user interfaces. Leveraging his expertise in technologies like Next.js, Rishaba focuses on delivering seamless digital experiences that blend aesthetics with functionality.
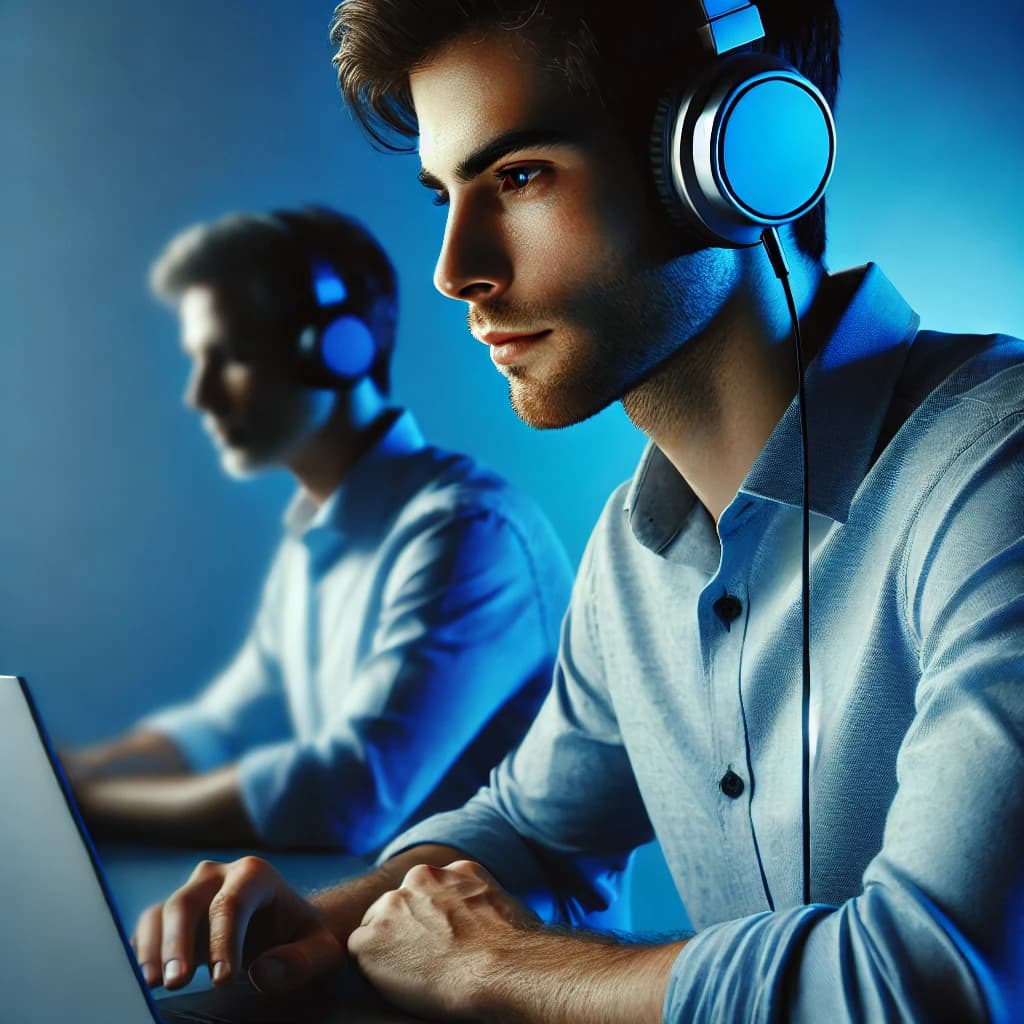