When working with databases in your application, ensuring type safety is critical to prevent errors and maintain clean, maintainable code. That’s where Kysely comes in—a type-safe and composable query builder that works beautifully with Nest.js.
In this post, we’ll walk you through integrating Kysely with Nest.js to build SQL queries in a type-safe, efficient, and maintainable way.
What is Kysely?
Kysely is a query builder library that helps you create SQL queries in a type-safe manner using a fluent API. With Kysely, you can avoid manual string concatenations, reduce the risk of SQL injection, and write cleaner code. Plus, its TypeScript support ensures your queries align perfectly with your database schema.
Check out the official Kysely documentation for more details.
Integrating Kysely with Nest.js
Using the nestjs-kysely
package, integrating Kysely into a Nest.js project is straightforward. Follow these steps to set up Kysely and start writing type-safe SQL queries in your application.
Step 1: Install Required Packages
Run the following command to install the necessary dependencies:
pnpm add nestjs-kysely kysely kysely-codegen pg
Step 2: Configure the Database
Create a .env
file in your project and add a DATABASE_URL
variable containing your database connection string:
DATABASE_URL='postgres://user:password@url:port/database'
This variable will be used to connect to your database and generate types from your schema.
Step 3: Add Kysely to Your App Module
Next, configure the Kysely module in your app.module.ts
file. Import the required modules and plugins, and set up the database connection:
import { Module } from '@nestjs/common';
import { KyselyModule } from 'nestjs-kysely';
import { CamelCasePlugin, PostgresDialect } from 'kysely';
import { Pool } from 'pg';
@Module({
imports: [
KyselyModule.forRoot({
dialect: new PostgresDialect({
pool: new Pool({
connectionString: process.env.DATABASE_URL,
ssl: {
rejectUnauthorized: false, // Adjust this based on your environment
},
}),
}),
plugins: [new CamelCasePlugin()], // Ensures column and table names are in camelCase
}),
],
})
export class AppModule {}
Step 4: Automate Type Generation
To leverage Kysely’s type-safe features, you’ll need to generate TypeScript types based on your database schema. Add the following script to your package.json
file:
"scripts": {
"build": "pnpm run k-types && nest build",
"k-types": "kysely-codegen --dialect postgres --camel-case"
}
Run this script to generate your database types:
pnpm run k-types
Step 5: Using Kysely in Your Services
Once the setup is complete, you can inject Kysely into your Nest.js services using the @InjectKysely
decorator. Here’s an example:
import { Injectable } from '@nestjs/common';
import { InjectKysely } from 'nestjs-kysely';
import { Kysely } from 'kysely';
import { DB } from 'kysely-codegen';
@Injectable()
export class UserService {
constructor(@InjectKysely() private readonly kdb: Kysely<DB>) {}
async getUsers() {
return this.kdb.selectFrom('users').selectAll().execute();
}
}
With this setup, you can write type-safe queries directly in your code. Kysely ensures that the queries you write match the structure of your database schema, catching errors at compile time instead of runtime.
Conclusion
By integrating Kysely with Nest.js, you can build type-safe SQL queries that are cleaner, easier to maintain, and free of common pitfalls. Whether you’re building simple queries or handling complex data operations, this setup ensures your database interactions remain robust and reliable.
We specialize in creating complex custom software solutions. If you’re looking for expert development services, feel free to reach out to us!
About Boopesh Mahendran
Boopesh is one of the Co-Founders of CyberMind Works and the Head of Engineering. An alum of Madras Institute of Technology with a rich professional background, he has previously worked at Adobe and Amazon. His expertise drives the innovative solutions at CyberMind Works.
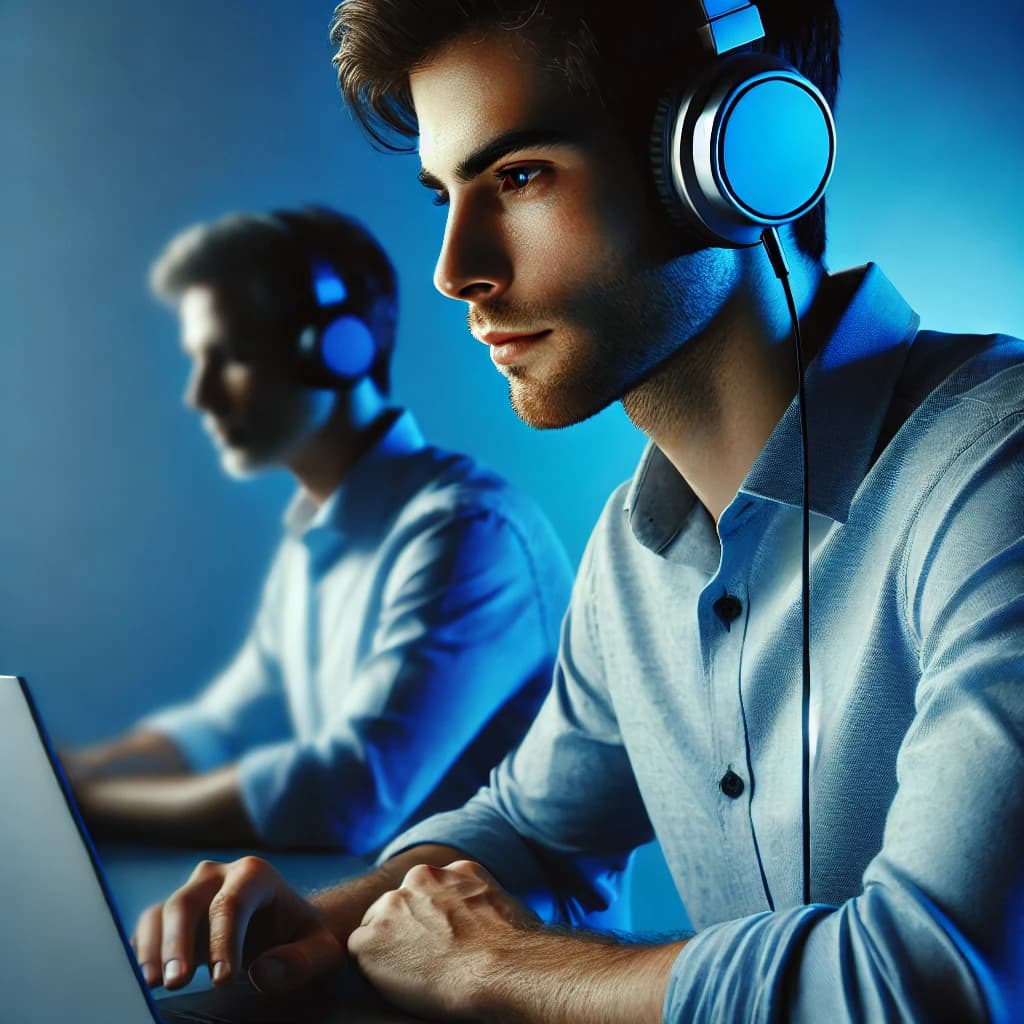