Infinite image carousels are a fantastic way to showcase content, from product images to portfolio galleries. They provide a seamless scrolling experience and enhance the visual appeal of web applications. This guide will show you how to build a high-performance, customizable infinite carousel in React using the lightweight Embla Carousel library.
Why Use Embla Carousel for React Carousels?
Embla Carousel is a modern and efficient choice for building carousels in React. Here’s why:
- Customizable: Offers flexibility to tailor the carousel to your design and functionality needs.
- Lightweight: Prioritizes performance without unnecessary bloat.
- Feature-Rich: Includes built-in support for infinite scrolling, smooth transitions, and more.
Step-by-Step Guide to Building an Infinite Carousel
Step 1: Install Embla Carousel
Start by installing the embla-carousel-react
package in your React project:
npm install embla-carousel-react
Step 2: Create a Basic Carousel
Let’s create a basic carousel that displays a series of items:
import React from "react";
import { useEmblaCarousel } from "embla-carousel-react";
const BasicCarousel = ({ items }: { items: string[] }) => {
const [emblaRef] = useEmblaCarousel();
return (
<div ref={emblaRef} style={{ overflow: "hidden", width: "100%" }}>
<div style={{ display: "flex" }}>
{items.map((item, idx) => (
<div key={idx} style={{ flex: "0 0 100%", padding: "10px", background: "lightgray" }}>
{item}
</div>
))}
</div>
</div>
);
};
export default BasicCarousel;
Key Features of the Code:
- Embla Reference (
emblaRef
): Connects the Embla instance to your carousel container. - Flexbox Layout: Ensures items are displayed in a horizontal row.
Step 3: Add Infinite Scrolling
To create an infinite carousel, enable the loop
option in Embla:
import React from "react";
import { useEmblaCarousel } from "embla-carousel-react";
const InfiniteCarousel = ({ items }: { items: string[] }) => {
const [emblaRef] = useEmblaCarousel({ loop: true });
return (
<div ref={emblaRef} style={{ overflow: "hidden", width: "100%" }}>
<div style={{ display: "flex" }}>
{items.map((item, idx) => (
<div key={idx} style={{ flex: "0 0 100%", padding: "10px", background: "lightblue" }}>
{item}
</div>
))}
</div>
</div>
);
};
export default InfiniteCarousel;
What’s New:
loop: true
: Enables infinite scrolling, allowing the carousel to seamlessly restart from the beginning.
Step 4: Add Navigation Controls
Enhance user interaction by adding "Previous" and "Next" buttons:
import React from "react";
import { useEmblaCarousel } from "embla-carousel-react";
const CarouselWithNavigation = ({ items }: { items: string[] }) => {
const [emblaRef, embla] = useEmblaCarousel({ loop: true });
const scrollPrev = () => embla?.scrollPrev();
const scrollNext = () => embla?.scrollNext();
return (
<div style={{ position: "relative" }}>
<div ref={emblaRef} style={{ overflow: "hidden", width: "100%" }}>
<div style={{ display: "flex" }}>
{items.map((item, idx) => (
<div key={idx} style={{ flex: "0 0 100%", padding: "10px", background: "lightblue" }}>
{item}
</div>
))}
</div>
</div>
<button onClick={scrollPrev} style={{ position: "absolute", left: "10px" }}>
Prev
</button>
<button onClick={scrollNext} style={{ position: "absolute", right: "10px" }}>
Next
</button>
</div>
);
};
export default CarouselWithNavigation;
Navigation Highlights:
- Use
scrollPrev
andscrollNext
methods for navigation. - Position buttons with CSS for a clean UI.
Advanced Customizations
1. Pagination Dots
Add pagination dots to indicate the current slide. Use Embla’s selectedScrollSnap
method to track active slides.
2. Autoplay
Create a seamless autoplay effect by calling scrollNext
on a timed interval:
import { useEffect } from "react";
const useAutoplay = (embla, interval = 3000) => {
useEffect(() => {
if (!embla) return;
const autoplay = setInterval(() => embla.scrollNext(), interval);
return () => clearInterval(autoplay);
}, [embla, interval]);
};
Performance Tips
- Lazy Loading: Use libraries like
react-lazyload
to defer loading of offscreen images. - Image Optimization: Convert images to formats like WebP and serve responsive sizes.
- Virtualization: Use
react-window
orreact-virtual
to render only visible slides. - Throttling Events: Debounce resize or scroll events to improve performance.
Embla Carousel vs. Other Libraries
Feature | Embla | Swiper | Slick Carousel |
---|---|---|---|
Lightweight | ✅ | ❌ (Heavier) | ❌ |
Customizable | ✅ | ✅ | ✅ |
Built-in Features | Basic (Modular) | Advanced (Autoplay, etc.) | Basic |
Performance | High | Medium | Low |
Further Reading
- Embla Carousel Documentation
- Optimizing Image Carousels
- React Lazy Loading
Conclusion
Building an infinite image carousel with Embla Carousel offers a perfect balance between performance and flexibility. With features like infinite scrolling, navigation controls, and customization options, Embla empowers developers to create engaging and dynamic carousels for modern web applications.
Ready to elevate your web experience with seamless carousels? Start with Embla Carousel today!
About Rishaba Priyan
Rishaba Priyan: Frontend Developer | Crafting Seamless User Experiences
At CyberMind Works, Rishaba Priyan excels as a Frontend Developer, specializing in creating intuitive and engaging user interfaces. Leveraging his expertise in technologies like Next.js, Rishaba focuses on delivering seamless digital experiences that blend aesthetics with functionality.
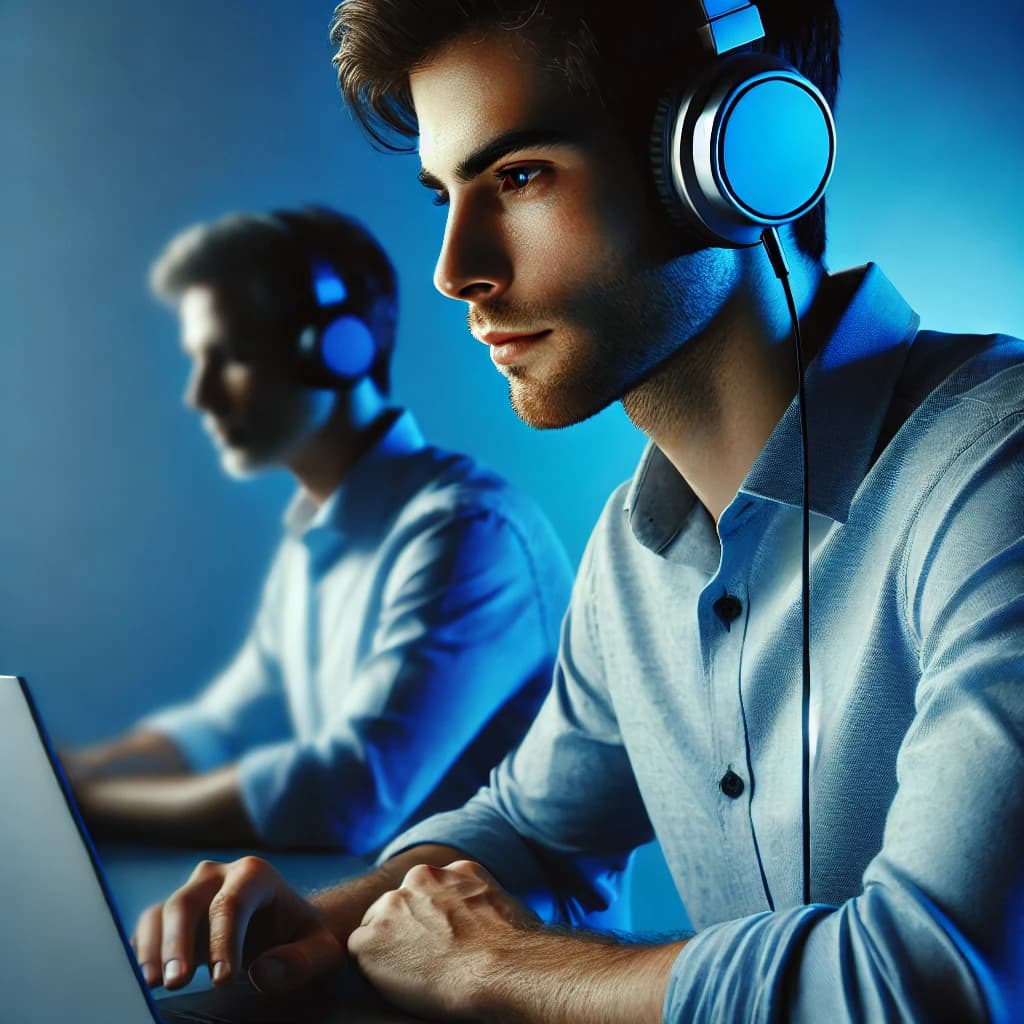