Image manipulation is a crucial feature in modern web applications, enabling users to crop and zoom images effortlessly. Whether it's for profile pictures, thumbnails, or detailed image exploration, these features significantly enhance user experience. In this guide, we’ll explore how to implement cropping with react-easy-crop
and zooming with react-medium-image-zoom
in React.
Why Add Image Manipulation Features?
- Improved User Experience: Allows users to customize images to their needs.
- Dynamic Content: Enables flexible visual editing for applications like e-commerce, social media, or design tools.
- Interactive Features: Zooming provides a detailed look at images, ideal for high-resolution visuals.
1. How to Implement Image Cropping in React
Why Use react-easy-crop
?
- Ease of Integration: Simple API for quick setup.
- Advanced Features: Includes zoom, rotation, and aspect ratio adjustments.
- Customizable: Adapts to a wide range of design requirements.
Step-by-Step Guide
Step 1: Install the Library
Run the following command to add react-easy-crop
to your project:
npm install react-easy-crop
Step 2: Create a Basic Cropper
import React, { useState } from "react";
import Cropper from "react-easy-crop";
const ImageCropper = ({ imageSrc }: { imageSrc: string }) => {
const [crop, setCrop] = useState({ x: 0, y: 0 });
const [zoom, setZoom] = useState(1);
return (
<div style={{ position: "relative", width: "100%", height: "400px" }}>
<Cropper
image={imageSrc}
crop={crop}
zoom={zoom}
aspect={1} // Maintains a square aspect ratio
onCropChange={setCrop}
onZoomChange={setZoom}
/>
</div>
);
};
export default ImageCropper;
Step 3: Save the Cropped Image
To save the cropped area, convert it into a Blob
using the canvas API:
utils/getCroppedImg.ts
:
import { Area } from "react-easy-crop";
const createImage = (url: string): Promise<HTMLImageElement> =>
new Promise((resolve, reject) => {
const img = new Image();
img.crossOrigin = "anonymous"; // Avoid CORS issues
img.onload = () => resolve(img);
img.onerror = reject;
img.src = url;
});
export default async function getCroppedImg(imageSrc: string, cropArea: Area): Promise<Blob> {
const img = await createImage(imageSrc);
const canvas = document.createElement("canvas");
const ctx = canvas.getContext("2d");
canvas.width = cropArea.width;
canvas.height = cropArea.height;
ctx?.drawImage(
img,
cropArea.x,
cropArea.y,
cropArea.width,
cropArea.height,
0,
0,
cropArea.width,
cropArea.height
);
return new Promise<Blob>((resolve) => {
canvas.toBlob(resolve, "image/jpeg");
});
}
Usage in the Cropper Component:
const handleSave = async () => {
if (croppedAreaPixels) {
const croppedImage = await getCroppedImg(imageSrc, croppedAreaPixels);
onSave(croppedImage);
}
};
2. How to Add Image Zooming in React
Why Use react-medium-image-zoom
?
- Simple Setup: Integrates easily into React applications.
- Interactive Experience: Provides intuitive zoom controls.
- Lightweight: Minimal impact on application performance.
Step-by-Step Guide
Step 1: Install the Library
Add react-medium-image-zoom
to your project:
npm install react-medium-image-zoom
Step 2: Create a Zoomable Image Component
import React from "react";
import Zoom from "react-medium-image-zoom";
import "react-medium-image-zoom/dist/styles.css";
const ZoomableImage = ({ src, alt }: { src: string; alt: string }) => (
<Zoom>
<img src={src} alt={alt} style={{ width: "100%", height: "auto" }} />
</Zoom>
);
export default ZoomableImage;
Step 3: Add Controlled Zooming
Control the zoom state programmatically to enhance user interactions:
import React, { useState } from "react";
import Zoom from "react-medium-image-zoom";
const ControlledZoomImage = ({ src, alt }: { src: string; alt: string }) => {
const [isZoomed, setIsZoomed] = useState(false);
return (
<Zoom isZoomed={isZoomed} onZoomChange={setIsZoomed}>
<img src={src} alt={alt} style={{ width: "100%", height: "auto" }} />
</Zoom>
);
};
export default ControlledZoomImage;
3. Combine Cropping and Zooming
You can use both cropping and zooming components together to build a complete image manipulation tool. For instance:
- Enable users to crop images first and then zoom into the cropped area for detailed editing.
- Add interactive features like saving or downloading the cropped image.
Best Practices for Image Manipulation
- CORS Handling: Ensure images are accessible by setting
crossOrigin="anonymous"
. - Lazy Loading: Load images asynchronously to improve performance.
- Responsive Design: Use flexible styles to make the components mobile-friendly.
Conclusion
Adding cropping and zooming capabilities to your React application enhances user experience and makes your app more interactive. With libraries like react-easy-crop
and react-medium-image-zoom
, implementing these features is straightforward. Start integrating these tools today to create engaging and functional image editing features in your React projects.
About Rishaba Priyan
Rishaba Priyan: Frontend Developer | Crafting Seamless User Experiences
At CyberMind Works, Rishaba Priyan excels as a Frontend Developer, specializing in creating intuitive and engaging user interfaces. Leveraging his expertise in technologies like Next.js, Rishaba focuses on delivering seamless digital experiences that blend aesthetics with functionality.
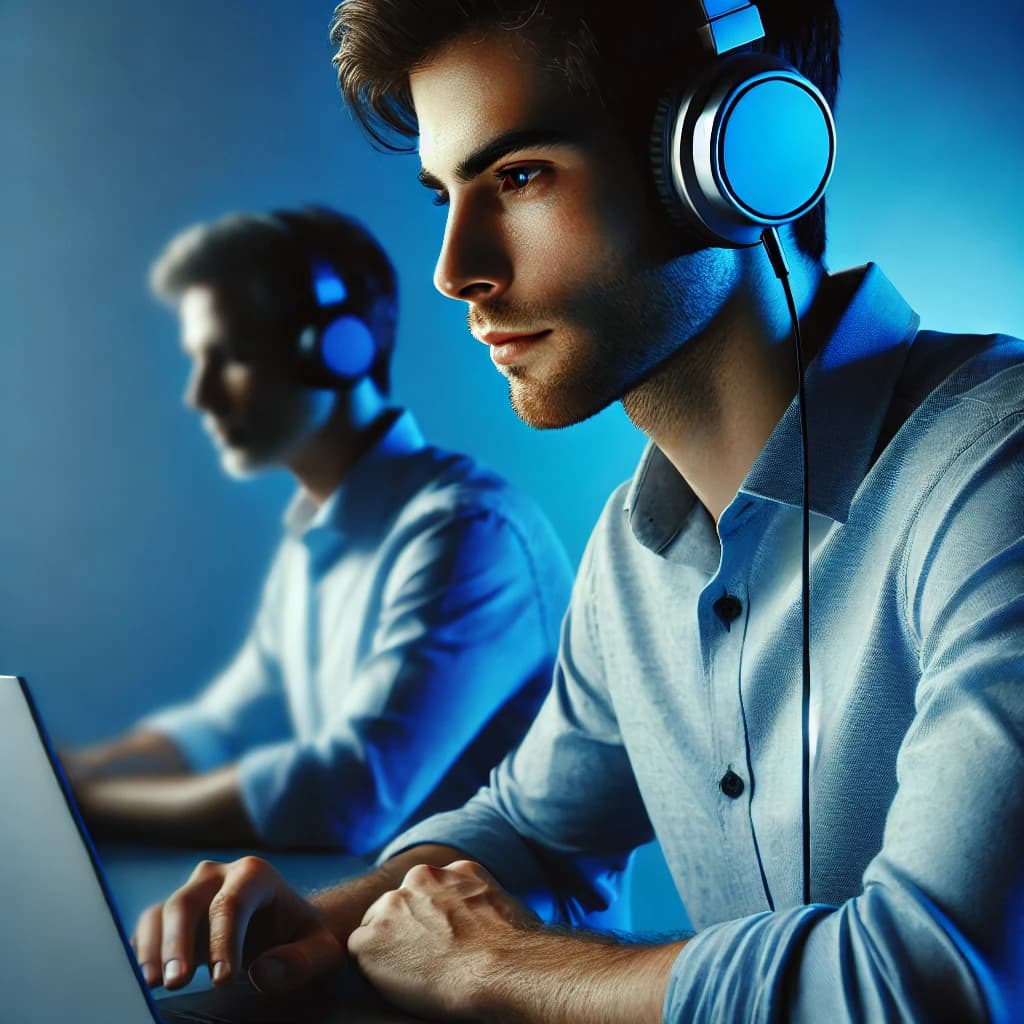