Images play a critical role in web performance and user experience, but they often contribute to large payload sizes that slow down page load times. To tackle this, Next.js offers an out-of-the-box solution with its Image
component, enabling powerful image optimization features such as resizing, lazy loading, modern format support, and dynamic caching.
This guide takes you through:
- How Next.js optimizes images under the hood.
- Responsive image techniques to improve performance.
- Implementing custom loaders for unique needs.
- Overcoming limitations of the Next.js
Image
component. - Exploring alternatives for advanced use cases.
By the end, you’ll be equipped with the strategies to deliver faster, more efficient, and visually appealing web experiences.
1. How Next.js Image Optimization Works
The Image
component in Next.js automates optimization, reducing developer effort while enhancing performance.
Under the Hood
- Dynamic Resizing and Compression:
- Images are resized to the requested dimensions, maintaining aspect ratios.
- Compression is applied using efficient formats like WebP for smaller file sizes.
- Lazy Loading:
- By default, images outside the viewport are not loaded until they’re needed, improving initial page load speed.
- Caching:
- Once optimized, images are cached for subsequent requests, reducing server load.
- Responsive Delivery:
- Next.js uses the
sizes
attribute to serve images tailored to the user’s viewport, minimizing unnecessary data transfer.
- Next.js uses the
Example Workflow:
- A user requests an image.
- The server resizes, compresses, and serves the optimized version.
- The optimized image is cached for future use.
2. Leveraging the Next.js Image
Component
The Image
component provides a seamless way to render optimized images.
Basic Usage
import Image from "next/image";
export default function Example() {
return (
<Image
src="/example.jpg"
alt="Example Image"
width={800}
height={600}
quality={80} // Optimized to 80% quality
/>
);
}
Responsive Images with sizes
The sizes
attribute ensures the right image size is delivered based on the user’s viewport:
<Image
src="/example.jpg"
alt="Responsive Image"
sizes="(max-width: 768px) 100vw, (max-width: 1200px) 50vw, 25vw"
width={1200}
height={800}
/>
How It Works:
- For viewports ≤ 768px: The image spans 100% of the screen.
- For viewports ≤ 1200px: It spans 50%.
- Beyond 1200px: It spans 25%.
This reduces bandwidth usage and ensures high performance across devices.
Fluid Containers with fill
For dynamic containers, use the fill
attribute to make images fit within their parent element:
<div style={{ position: "relative", width: "100%", height: "400px" }}>
<Image
src="/example.jpg"
alt="Dynamic Image"
fill
style={{ objectFit: "cover" }}
/>
</div>
3. Extending Functionality with Custom Loaders
Custom loaders allow you to integrate external image services or add unique transformations.
Example: Integrating wsrv.nl
wsrv.nl is a CDN-based service that resizes, compresses, and delivers optimized images dynamically.
Custom Loader Setup
import { ImageLoaderProps } from "next/image";
const wsrvLoader = ({ src, width, quality }: ImageLoaderProps) => {
const encodedSrc = encodeURIComponent(src);
return `https://wsrv.nl/?url=${encodedSrc}&w=${width}&q=${quality || 75}`;
};
Using the Custom Loader
<Image
loader={wsrvLoader}
src="/example.jpg"
alt="Custom Loader Example"
width={800}
height={600}
quality={90}
/>
4. Advanced Responsive Image Techniques
Next.js handles responsive images efficiently using attributes like srcSet
and sizes
. Let’s take it further:
How It Works
srcSet
: Contains URLs for various image resolutions.sizes
: Determines the display size relative to the viewport.
Example
<Image
src="/example.jpg"
sizes="(max-width: 768px) 100vw, 50vw"
width={1000}
height={600}
/>
For smaller screens, the browser downloads a smaller image, ensuring efficient data usage.
5. Overcoming Limitations of Next.js Image
Despite its strengths, the Image
component has limitations:
1. Server-Side Overhead
Image processing on the server can strain resources under high traffic.
Solution: Use CDNs to offload optimization tasks.
2. Incompatibility with Static Exports
The Image
component doesn’t work with next export
.
Solution: Pair with loaders like Cloudinary or wsrv.nl for pre-optimized images.
3. Limited Transformations
Advanced editing like cropping or applying filters requires external tools.
Solution: Use tools like ImageKit or Cloudinary for such transformations.
6. Alternatives to Next.js Image Optimization
For more specialized needs, consider these alternatives:
- ImageKit:
- Real-time transformations and AI-based optimizations.
- Works seamlessly with modern frameworks.
- Cloudinary:
- Comprehensive media management.
- Powerful APIs for dynamic cropping, overlays, and effects.
- Manual Optimization:
- Tools like Squoosh or TinyPNG for pre-deployment image compression.
7. Optimizing for Core Web Vitals
Largest Contentful Paint (LCP)
- Use
priority
for hero images to load them faster. - Deliver images in modern formats like WebP.
Cumulative Layout Shift (CLS)
- Define
width
andheight
attributes for all images. - Use CSS aspect ratio boxes for responsiveness.
First Input Delay (FID)
- Reduce payload size with lazy loading and optimized images.
Conclusion
Image optimization in Next.js goes beyond just saving bandwidth—it enhances load times, improves SEO, and creates a better user experience. By mastering the Image
component, extending functionality with custom loaders, and leveraging modern tools like wsrv.nl or Cloudinary, you can deliver visually stunning and highly performant web applications.
Ready to build blazing-fast web apps? Contact Cybermindworks and bring your vision to life with expert solutions tailored to your needs.
Further Reading
About Rishaba Priyan
Rishaba Priyan: Frontend Developer | Crafting Seamless User Experiences
At CyberMind Works, Rishaba Priyan excels as a Frontend Developer, specializing in creating intuitive and engaging user interfaces. Leveraging his expertise in technologies like Next.js, Rishaba focuses on delivering seamless digital experiences that blend aesthetics with functionality.
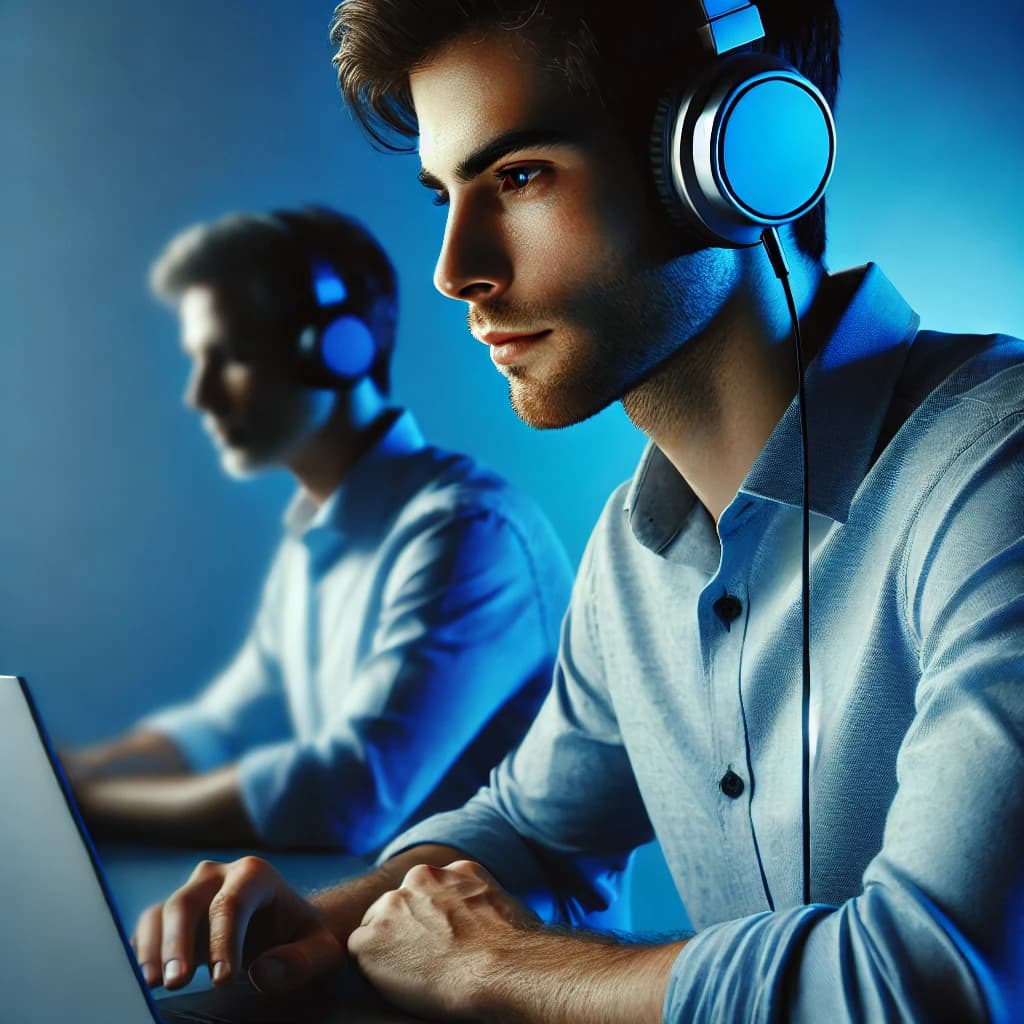